* Before you open a ticket or send a pull request, [search](https://github.com/jashkenas/coffee-script/issues) for previous discussions about the same feature or issue. Add to the earlier ticket if you find one.
* Before you open a ticket or send a pull request, [search](https://github.com/jashkenas/coffeescript/issues) for previous discussions about the same feature or issue. Add to the earlier ticket if you find one.
* Before sending a pull request for a feature, be sure to have [tests](https://github.com/jashkenas/coffee-script/tree/master/test).
* Before sending a pull request for a feature, be sure to have [tests](https://github.com/jashkenas/coffeescript/tree/master/test).
* Use the same coding style as the rest of the [codebase](https://github.com/jashkenas/coffee-script/tree/master/src). If you're just getting started with CoffeeScript, there's a nice [style guide](https://github.com/polarmobile/coffeescript-style-guide).
* Use the same coding style as the rest of the [codebase](https://github.com/jashkenas/coffeescript/tree/master/src). If you're just getting started with CoffeeScript, there's a nice [style guide](https://github.com/polarmobile/coffeescript-style-guide).
* In your pull request, do not add documentation to `index.html` or re-build the minified `coffee-script.js` file. We'll do those things before cutting a new release.
errorText=errorToken===tokens[tokens.length-1]?'end of input':errorTag==='INDENT'||errorTag==='OUTDENT'?'indentation':helpers.nameWhitespaceCharacter(errorText);
BANNER='Usage: coffee [options] path/to/script.coffee -- [args]\n\nIf called without options, `coffee` will run your script.';
SWITCHES=[['-b','--bare','compile without a top-level function wrapper'],['-c','--compile','compile to JavaScript and save as .js files'],['-e','--eval','pass a string from the command line as input'],['-h','--help','display this help message'],['-i','--interactive','run an interactive CoffeeScript REPL'],['-j','--join [FILE]','concatenate the source CoffeeScript before compiling'],['-m','--map','generate source map and save as .map files'],['-n','--nodes','print out the parse tree that the parser produces'],['--nodejs [ARGS]','pass options directly to the "node" binary'],['--no-header','suppress the "Generated by" header'],['-o','--output [DIR]','set the output directory for compiled JavaScript'],['-p','--print','print out the compiled JavaScript'],['-s','--stdio','listen for and compile scripts over stdio'],['-l','--literate','treat stdio as literate style coffee-script'],['-t','--tokens','print out the tokens that the lexer/rewriter produce'],['-v','--version','display the version number'],['-w','--watch','watch scripts for changes and rerun commands']];
SWITCHES=[['-b','--bare','compile without a top-level function wrapper'],['-c','--compile','compile to JavaScript and save as .js files'],['-e','--eval','pass a string from the command line as input'],['-h','--help','display this help message'],['-i','--interactive','run an interactive CoffeeScript REPL'],['-j','--join [FILE]','concatenate the source CoffeeScript before compiling'],['-m','--map','generate source map and save as .js.map files'],['-n','--nodes','print out the parse tree that the parser produces'],['--nodejs [ARGS]','pass options directly to the "node" binary'],['--no-header','suppress the "Generated by" header'],['-o','--output [DIR]','set the output directory for compiled JavaScript'],['-p','--print','print out the compiled JavaScript'],['-s','--stdio','listen for and compile scripts over stdio'],['-l','--literate','treat stdio as literate style coffee-script'],['-t','--tokens','print out the tokens that the lexer/rewriter produce'],['-v','--version','display the version number'],['-w','--watch','watch scripts for changes and rerun commands']];
opts={};
...
...
@@ -86,6 +86,7 @@
}
if(opts.join){
opts.join=path.resolve(opts.join);
console.error('\nThe --join option is deprecated and will be removed in a future version.\n\nIf for some reason it\'s necessary to share local variables between files,\nreplace...\n\n $ coffee --compile --join bundle.js -- a.coffee b.coffee c.coffee\n\nwith...\n\n $ cat a.coffee b.coffee c.coffee | coffee --compile --stdio > bundle.js\n');
"readme":"# qs\n\nA querystring parsing and stringifying library with some added security.\n\n[](http://travis-ci.org/hapijs/qs)\n\nLead Maintainer: [Nathan LaFreniere](https://github.com/nlf)\n\nThe **qs** module was originally created and maintained by [TJ Holowaychuk](https://github.com/visionmedia/node-querystring).\n\n## Usage\n\n```javascript\nvar Qs = require('qs');\n\nvar obj = Qs.parse('a=c'); // { a: 'c' }\nvar str = Qs.stringify(obj); // 'a=c'\n```\n\n### Parsing Objects\n\n```javascript\nQs.parse(string, [depth], [delimiter]);\n```\n\n**qs** allows you to create nested objects within your query strings, by surrounding the name of sub-keys with square brackets `[]`.\nFor example, the string `'foo[bar]=baz'` converts to:\n\n```javascript\n{\n foo: {\n bar: 'baz'\n }\n}\n```\n\nURI encoded strings work too:\n\n```javascript\nQs.parse('a%5Bb%5D=c');\n// { a: { b: 'c' } }\n```\n\nYou can also nest your objects, like `'foo[bar][baz]=foobarbaz'`:\n\n```javascript\n{\n foo: {\n bar: {\n baz: 'foobarbaz'\n }\n }\n}\n```\n\nBy default, when nesting objects **qs** will only parse up to 5 children deep. This means if you attempt to parse a string like\n`'a[b][c][d][e][f][g][h][i]=j'` your resulting object will be:\n\n```javascript\n{\n a: {\n b: {\n c: {\n d: {\n e: {\n f: {\n '[g][h][i]': 'j'\n }\n }\n }\n }\n }\n }\n}\n```\n\nThis depth can be overridden by passing a `depth` option to `Qs.parse(string, depth)`:\n\n```javascript\nQs.parse('a[b][c][d][e][f][g][h][i]=j', 1);\n// { a: { b: { '[c][d][e][f][g][h][i]': 'j' } } }\n```\n\nThe depth limit mitigate abuse when **qs** is used to parse user input, and it is recommended to keep it a reasonably small number.\n\nAn optional delimiter can also be passed:\n\n```javascript\nQs.parse('a=b;c=d', ';');\n// { a: 'b', c: 'd' }\n```\n\n### Parsing Arrays\n\n**qs** can also parse arrays using a similar `[]` notation:\n\n```javascript\nQs.parse('a[]=b&a[]=c');\n// { a: ['b', 'c'] }\n```\n\nYou may specify an index as well:\n\n```javascript\nQs.parse('a[1]=c&a[0]=b');\n// { a: ['b', 'c'] }\n```\n\nNote that the only difference between an index in an array and a key in an object is that the value between the brackets must be a number\nto create an array. When creating arrays with specific indices, **qs** will compact a sparse array to only the existing values preserving\ntheir order:\n\n```javascript\nQs.parse('a[1]=b&a[15]=c');\n// { a: ['b', 'c'] }\n```\n\nNote that an empty string is also a value, and will be preserved:\n\n```javascript\nQs.parse('a[]=&a[]=b');\n// { a: ['', 'b'] }\nQs.parse('a[0]=b&a[1]=&a[2]=c');\n// { a: ['b', '', 'c'] }\n```\n\n**qs** will also limit specifying indices in an array to a maximum index of `20`. Any array members with an index of greater than `20` will\ninstead be converted to an object with the index as the key:\n\n```javascript\nQs.parse('a[100]=b');\n// { a: { '100': 'b' } }\n```\n\nIf you mix notations, **qs** will merge the two items into an object:\n\n```javascript\nQs.parse('a[0]=b&a[b]=c');\n// { a: { '0': 'b', b: 'c' } }\n```\n\nYou can also create arrays of objects:\n\n```javascript\nQs.parse('a[][b]=c');\n// { a: [{ b: 'c' }] }\n```\n\n### Stringifying\n\n```javascript\nQs.stringify(object, [delimiter]);\n```\n\nWhen stringifying, **qs** always URI encodes output. Objects are stringified as you would expect:\n\n```javascript\nQs.stringify({ a: 'b' });\n// 'a=b'\nQs.stringify({ a: { b: 'c' } });\n// 'a%5Bb%5D=c'\n```\n\nExamples beyond this point will be shown as though the output is not URI encoded for clarity. Please note that the return values in these cases *will* be URI encoded during real usage.\n\nWhen arrays are stringified, they are always given explicit indices:\n\n```javascript\nQs.stringify({ a: ['b', 'c', 'd'] });\n// 'a[0]=b&a[1]=c&a[2]=d'\n```\n\nEmpty strings and null values will omit the value, but the equals sign (=) remains in place:\n\n```javascript\nQs.stringify({ a: '' });\n// 'a='\n```\n\nProperties that are set to `undefined` will be omitted entirely:\n\n```javascript\nQs.stringify({ a: null, b: undefined });\n// 'a='\n```\n\nThe delimiter may be overridden with stringify as well:\n\n```javascript\nQs.stringify({ a: 'b', c: 'd' }, ';');\n// 'a=b;c=d'\n```\n",
"readme":"# qs\n\nA querystring parsing and stringifying library with some added security.\n\n[](http://travis-ci.org/hapijs/qs)\n\nLead Maintainer: [Nathan LaFreniere](https://github.com/nlf)\n\nThe **qs** module was originally created and maintained by [TJ Holowaychuk](https://github.com/visionmedia/node-querystring).\n\n## Usage\n\n```javascript\nvar Qs = require('qs');\n\nvar obj = Qs.parse('a=c'); // { a: 'c' }\nvar str = Qs.stringify(obj); // 'a=c'\n```\n\n### Parsing Objects\n\n```javascript\nQs.parse(string, [options]);\n```\n\n**qs** allows you to create nested objects within your query strings, by surrounding the name of sub-keys with square brackets `[]`.\nFor example, the string `'foo[bar]=baz'` converts to:\n\n```javascript\n{\n foo: {\n bar: 'baz'\n }\n}\n```\n\nURI encoded strings work too:\n\n```javascript\nQs.parse('a%5Bb%5D=c');\n// { a: { b: 'c' } }\n```\n\nYou can also nest your objects, like `'foo[bar][baz]=foobarbaz'`:\n\n```javascript\n{\n foo: {\n bar: {\n baz: 'foobarbaz'\n }\n }\n}\n```\n\nBy default, when nesting objects **qs** will only parse up to 5 children deep. This means if you attempt to parse a string like\n`'a[b][c][d][e][f][g][h][i]=j'` your resulting object will be:\n\n```javascript\n{\n a: {\n b: {\n c: {\n d: {\n e: {\n f: {\n '[g][h][i]': 'j'\n }\n }\n }\n }\n }\n }\n}\n```\n\nThis depth can be overridden by passing a `depth` option to `Qs.parse(string, [options])`:\n\n```javascript\nQs.parse('a[b][c][d][e][f][g][h][i]=j', { depth: 1 });\n// { a: { b: { '[c][d][e][f][g][h][i]': 'j' } } }\n```\n\nThe depth limit helps mitigate abuse when **qs** is used to parse user input, and it is recommended to keep it a reasonably small number.\n\nFor similar reasons, by default **qs** will only parse up to 1000 parameters. This can be overridden by passing a `parameterLimit` option:\n\n```javascript\nQs.parse('a=b&c=d', { parameterLimit: 1 });\n// { a: 'b' }\n```\n\nAn optional delimiter can also be passed:\n\n```javascript\nQs.parse('a=b;c=d', { delimiter: ';' });\n// { a: 'b', c: 'd' }\n```\n\nDelimiters can be a regular expression too:\n\n```javascript\nQs.parse('a=b;c=d,e=f', { delimiter: /[;,]/ });\n// { a: 'b', c: 'd', e: 'f' }\n```\n\n### Parsing Arrays\n\n**qs** can also parse arrays using a similar `[]` notation:\n\n```javascript\nQs.parse('a[]=b&a[]=c');\n// { a: ['b', 'c'] }\n```\n\nYou may specify an index as well:\n\n```javascript\nQs.parse('a[1]=c&a[0]=b');\n// { a: ['b', 'c'] }\n```\n\nNote that the only difference between an index in an array and a key in an object is that the value between the brackets must be a number\nto create an array. When creating arrays with specific indices, **qs** will compact a sparse array to only the existing values preserving\ntheir order:\n\n```javascript\nQs.parse('a[1]=b&a[15]=c');\n// { a: ['b', 'c'] }\n```\n\nNote that an empty string is also a value, and will be preserved:\n\n```javascript\nQs.parse('a[]=&a[]=b');\n// { a: ['', 'b'] }\nQs.parse('a[0]=b&a[1]=&a[2]=c');\n// { a: ['b', '', 'c'] }\n```\n\n**qs** will also limit specifying indices in an array to a maximum index of `20`. Any array members with an index of greater than `20` will\ninstead be converted to an object with the index as the key:\n\n```javascript\nQs.parse('a[100]=b');\n// { a: { '100': 'b' } }\n```\n\nThis limit can be overridden by passing an `arrayLimit` option:\n\n```javascript\nQs.parse('a[1]=b', { arrayLimit: 0 });\n// { a: { '1': 'b' } }\n```\n\nIf you mix notations, **qs** will merge the two items into an object:\n\n```javascript\nQs.parse('a[0]=b&a[b]=c');\n// { a: { '0': 'b', b: 'c' } }\n```\n\nYou can also create arrays of objects:\n\n```javascript\nQs.parse('a[][b]=c');\n// { a: [{ b: 'c' }] }\n```\n\n### Stringifying\n\n```javascript\nQs.stringify(object, [options]);\n```\n\nWhen stringifying, **qs** always URI encodes output. Objects are stringified as you would expect:\n\n```javascript\nQs.stringify({ a: 'b' });\n// 'a=b'\nQs.stringify({ a: { b: 'c' } });\n// 'a%5Bb%5D=c'\n```\n\nExamples beyond this point will be shown as though the output is not URI encoded for clarity. Please note that the return values in these cases *will* be URI encoded during real usage.\n\nWhen arrays are stringified, they are always given explicit indices:\n\n```javascript\nQs.stringify({ a: ['b', 'c', 'd'] });\n// 'a[0]=b&a[1]=c&a[2]=d'\n```\n\nEmpty strings and null values will omit the value, but the equals sign (=) remains in place:\n\n```javascript\nQs.stringify({ a: '' });\n// 'a='\n```\n\nProperties that are set to `undefined` will be omitted entirely:\n\n```javascript\nQs.stringify({ a: null, b: undefined });\n// 'a='\n```\n\nThe delimiter may be overridden with stringify as well:\n\n```javascript\nQs.stringify({ a: 'b', c: 'd' }, { delimiter: ';' });\n// 'a=b;c=d'\n```\n",
"readme":"[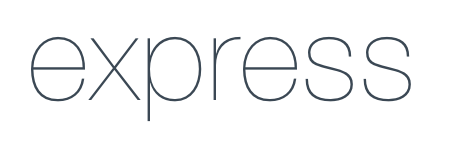](https://expressjs.com/)\n\n Fast, unopinionated, minimalist web framework for [node](http://nodejs.org).\n\n [](https://www.npmjs.org/package/express)\n [](https://travis-ci.org/strongloop/express)\n [](https://coveralls.io/r/strongloop/express)\n [](https://www.gittip.com/dougwilson/)\n\n```js\nvar express = require('express')\nvar app = express()\n\napp.get('/', function (req, res) {\n res.send('Hello World')\n})\n\napp.listen(3000)\n```\n\n **PROTIP** Be sure to read [Migrating from 3.x to 4.x](https://github.com/strongloop/express/wiki/Migrating-from-3.x-to-4.x) as well as [New features in 4.x](https://github.com/strongloop/express/wiki/New-features-in-4.x).\n\n### Installation\n\n```bash\n$ npm install express\n```\n\n## Quick Start\n\n The quickest way to get started with express is to utilize the executable [`express(1)`](https://github.com/expressjs/generator) to generate an application as shown below:\n\n Install the executable. The executable's major version will match Express's:\n\n```bash\n$ npm install -g express-generator@4\n```\n\n Create the app:\n\n```bash\n$ express /tmp/foo && cd /tmp/foo\n```\n\n Install dependencies:\n\n```bash\n$ npm install\n```\n\n Start the server:\n\n```bash\n$ npm start\n```\n\n## Features\n\n * Robust routing\n * HTTP helpers (redirection, caching, etc)\n * View system supporting 14+ template engines\n * Content negotiation\n * Focus on high performance\n * Executable for generating applications quickly\n * High test coverage\n\n## Philosophy\n\n The Express philosophy is to provide small, robust tooling for HTTP servers, making\n it a great solution for single page applications, web sites, hybrids, or public\n HTTP APIs.\n\n Express does not force you to use any specific ORM or template engine. With support for over\n 14 template engines via [Consolidate.js](https://github.com/visionmedia/consolidate.js),\n you can quickly craft your perfect framework.\n\n## More Information\n\n * [Website and Documentation](http://expressjs.com/) - [[website repo](https://github.com/strongloop/expressjs.com)]\n * [Github Organization](https://github.com/expressjs) for Official Middleware & Modules\n * [#express](https://webchat.freenode.net/?channels=express) on freenode IRC\n * Visit the [Wiki](https://github.com/strongloop/express/wiki)\n * [Google Group](https://groups.google.com/group/express-js) for discussion\n * [Русскоязычная документация](http://jsman.ru/express/)\n * [한국어 문서](http://expressjs.kr) - [[website repo](https://github.com/Hanul/expressjs.kr)]\n * Run express examples [online](https://runnable.com/express)\n\n## Viewing Examples\n\n Clone the Express repo, then install the dev dependencies to install all the example / test suite dependencies:\n\n```bash\n$ git clone git://github.com/strongloop/express.git --depth 1\n$ cd express\n$ npm install\n```\n\n Then run whichever example you want:\n\n $ node examples/content-negotiation\n\n You can also view live examples here:\n\n <a href=\"https://runnable.com/express\" target=\"_blank\"><img src=\"https://runnable.com/external/styles/assets/runnablebtn.png\" style=\"width:67px;height:25px;\"></a>\n\n## Running Tests\n\n To run the test suite, first invoke the following command within the repo, installing the development dependencies:\n\n```bash\n$ npm install\n```\n\n Then run the tests:\n\n```bash\n$ npm test\n```\n\n### Contributors\n\n * Author: [TJ Holowaychuk](https://github.com/visionmedia)\n * Lead Maintainer: [Douglas Christopher Wilson](https://github.com/dougwilson)\n * [All Contributors](https://github.com/strongloop/express/graphs/contributors)\n\n### License\n\n [MIT](LICENSE)\n",
"readme":"[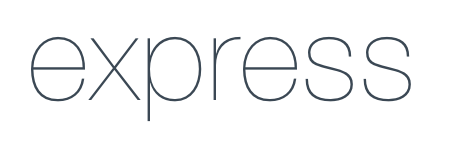](http://expressjs.com/)\n\n Fast, unopinionated, minimalist web framework for [node](http://nodejs.org).\n\n [](https://www.npmjs.org/package/express)\n [](https://travis-ci.org/strongloop/express)\n [](https://coveralls.io/r/strongloop/express)\n [](https://www.gittip.com/dougwilson/)\n\n```js\nvar express = require('express')\nvar app = express()\n\napp.get('/', function (req, res) {\n res.send('Hello World')\n})\n\napp.listen(3000)\n```\n\n **PROTIP** Be sure to read [Migrating from 3.x to 4.x](https://github.com/strongloop/express/wiki/Migrating-from-3.x-to-4.x) as well as [New features in 4.x](https://github.com/strongloop/express/wiki/New-features-in-4.x).\n\n### Installation\n\n```bash\n$ npm install express\n```\n\n## Quick Start\n\n The quickest way to get started with express is to utilize the executable [`express(1)`](https://github.com/expressjs/generator) to generate an application as shown below:\n\n Install the executable. The executable's major version will match Express's:\n\n```bash\n$ npm install -g express-generator@4\n```\n\n Create the app:\n\n```bash\n$ express /tmp/foo && cd /tmp/foo\n```\n\n Install dependencies:\n\n```bash\n$ npm install\n```\n\n Start the server:\n\n```bash\n$ npm start\n```\n\n## Features\n\n * Robust routing\n * HTTP helpers (redirection, caching, etc)\n * View system supporting 14+ template engines\n * Content negotiation\n * Focus on high performance\n * Executable for generating applications quickly\n * High test coverage\n\n## Philosophy\n\n The Express philosophy is to provide small, robust tooling for HTTP servers, making\n it a great solution for single page applications, web sites, hybrids, or public\n HTTP APIs.\n\n Express does not force you to use any specific ORM or template engine. With support for over\n 14 template engines via [Consolidate.js](https://github.com/visionmedia/consolidate.js),\n you can quickly craft your perfect framework.\n\n## More Information\n\n * [Website and Documentation](http://expressjs.com/) - [[website repo](https://github.com/strongloop/expressjs.com)]\n * [Github Organization](https://github.com/expressjs) for Official Middleware & Modules\n * [#express](https://webchat.freenode.net/?channels=express) on freenode IRC\n * Visit the [Wiki](https://github.com/strongloop/express/wiki)\n * [Google Group](https://groups.google.com/group/express-js) for discussion\n * [Русскоязычная документация](http://jsman.ru/express/)\n * [한국어 문서](http://expressjs.kr) - [[website repo](https://github.com/Hanul/expressjs.kr)]\n * Run express examples [online](https://runnable.com/express)\n\n## Viewing Examples\n\n Clone the Express repo, then install the dev dependencies to install all the example / test suite dependencies:\n\n```bash\n$ git clone git://github.com/strongloop/express.git --depth 1\n$ cd express\n$ npm install\n```\n\n Then run whichever example you want:\n\n $ node examples/content-negotiation\n\n You can also view live examples here:\n\n <a href=\"https://runnable.com/express\" target=\"_blank\"><img src=\"https://runnable.com/external/styles/assets/runnablebtn.png\" style=\"width:67px;height:25px;\"></a>\n\n## Running Tests\n\n To run the test suite, first invoke the following command within the repo, installing the development dependencies:\n\n```bash\n$ npm install\n```\n\n Then run the tests:\n\n```bash\n$ npm test\n```\n\n### Contributors\n\n * Author: [TJ Holowaychuk](https://github.com/visionmedia)\n * Lead Maintainer: [Douglas Christopher Wilson](https://github.com/dougwilson)\n * [All Contributors](https://github.com/strongloop/express/graphs/contributors)\n\n### License\n\n [MIT](LICENSE)\n",